224. Basic Calculator LeetCode Solution
In this guide, you will get 224. Basic Calculator LeetCode Solution with the best time and space complexity. The solution to Basic Calculator problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Basic Calculator solution in C++
- Basic Calculator solution in Java
- Basic Calculator solution in Python
- Additional Resources
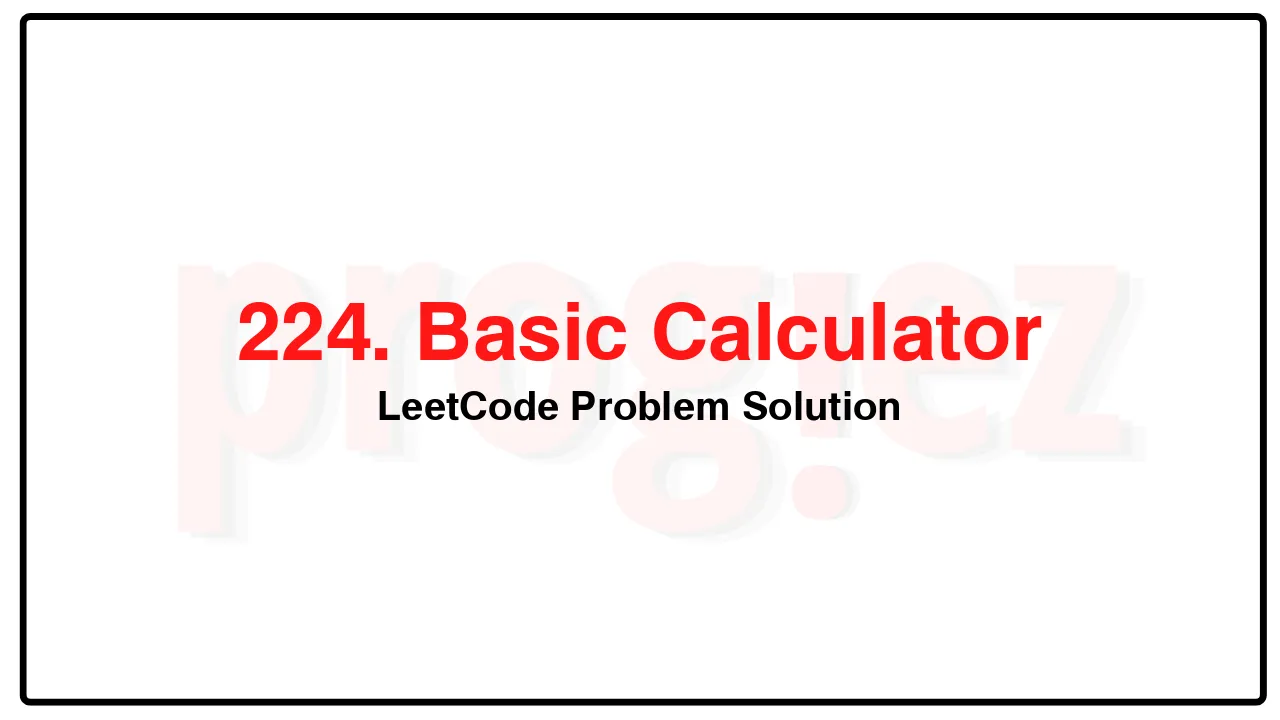
Problem Statement of Basic Calculator
Given a string s representing a valid expression, implement a basic calculator to evaluate it, and return the result of the evaluation.
Note: You are not allowed to use any built-in function which evaluates strings as mathematical expressions, such as eval().
Example 1:
Input: s = “1 + 1″
Output: 2
Example 2:
Input: s = ” 2-1 + 2 ”
Output: 3
Example 3:
Input: s = “(1+(4+5+2)-3)+(6+8)”
Output: 23
Constraints:
1 <= s.length <= 3 * 105
s consists of digits, '+', '-', '(', ')', and ' '.
s represents a valid expression.
'+' is not used as a unary operation (i.e., "+1" and "+(2 + 3)" is invalid).
'-' could be used as a unary operation (i.e., "-1" and "-(2 + 3)" is valid).
There will be no two consecutive operators in the input.
Every number and running calculation will fit in a signed 32-bit integer.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(n)
224. Basic Calculator LeetCode Solution in C++
class Solution {
public:
int calculate(string s) {
int ans = 0;
int num = 0;
int sign = 1;
stack<int> stack{{sign}}; // stack.top() := the current environment's sign
for (const char c : s)
if (isdigit(c))
num = num * 10 + (c - '0');
else if (c == '(')
stack.push(sign);
else if (c == ')')
stack.pop();
else if (c == '+' || c == '-') {
ans += sign * num;
sign = (c == '+' ? 1 : -1) * stack.top();
num = 0;
}
return ans + sign * num;
}
};
/* code provided by PROGIEZ */
224. Basic Calculator LeetCode Solution in Java
class Solution {
public int calculate(String s) {
int ans = 0;
int num = 0;
int sign = 1;
// stack.peek() := the current environment's sign
Deque<Integer> stack = new ArrayDeque<>();
stack.push(sign);
for (final char c : s.toCharArray())
if (Character.isDigit(c))
num = num * 10 + (c - '0');
else if (c == '(')
stack.push(sign);
else if (c == ')')
stack.pop();
else if (c == '+' || c == '-') {
ans += sign * num;
sign = (c == '+' ? 1 : -1) * stack.peek();
num = 0;
}
return ans + sign * num;
}
}
// code provided by PROGIEZ
224. Basic Calculator LeetCode Solution in Python
class Solution:
def calculate(self, s: str) -> int:
ans = 0
num = 0
sign = 1
stack = [sign] # stack[-1]: the current environment's sign
for c in s:
if c.isdigit():
num = num * 10 + int(c)
elif c == '(':
stack.append(sign)
elif c == ')':
stack.pop()
elif c == '+' or c == '-':
ans += sign * num
sign = (1 if c == '+' else -1) * stack[-1]
num = 0
return ans + sign * num
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.