61. Rotate List LeetCode Solution
In this guide, you will get 61. Rotate List LeetCode Solution with the best time and space complexity. The solution to Rotate List problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Rotate List solution in C++
- Rotate List solution in Java
- Rotate List solution in Python
- Additional Resources
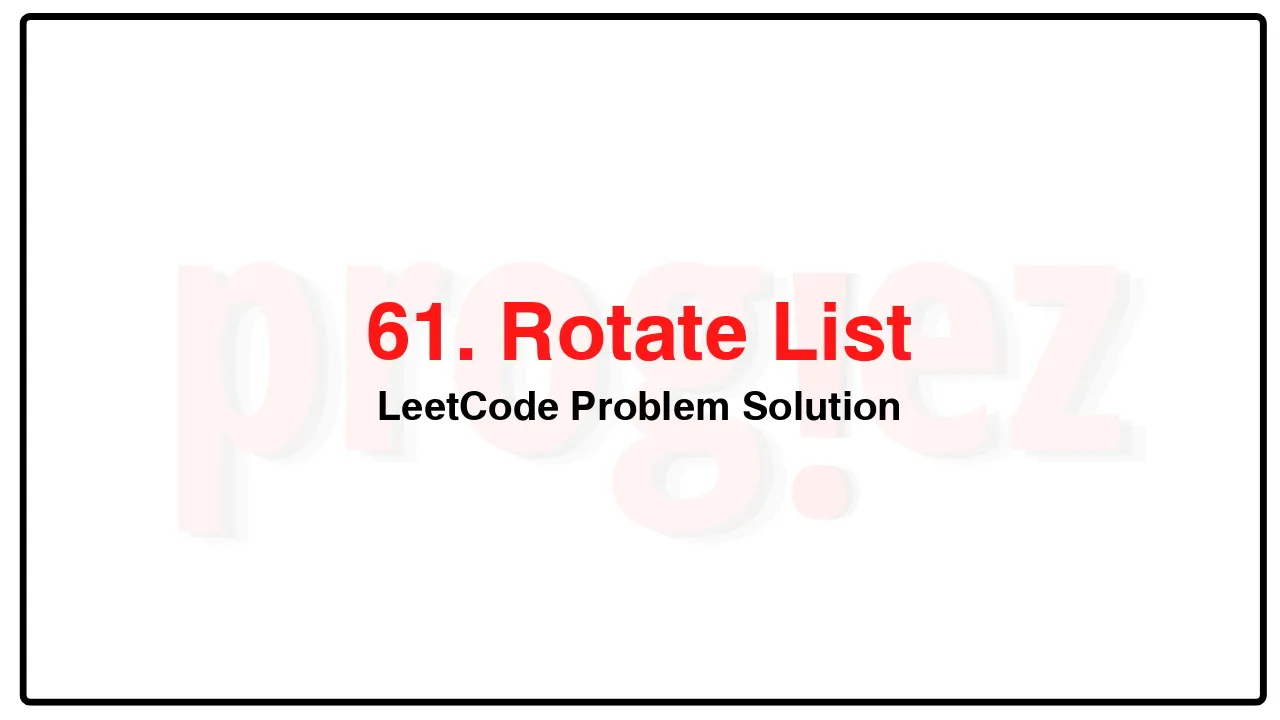
Problem Statement of Rotate List
Given the head of a linked list, rotate the list to the right by k places.
Example 1:
Input: head = [1,2,3,4,5], k = 2
Output: [4,5,1,2,3]
Example 2:
Input: head = [0,1,2], k = 4
Output: [2,0,1]
Constraints:
The number of nodes in the list is in the range [0, 500].
-100 <= Node.val <= 100
0 <= k <= 2 * 109
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
61. Rotate List LeetCode Solution in C++
class Solution {
public:
ListNode* rotateRight(ListNode* head, int k) {
if (!head || !head->next || k == 0)
return head;
ListNode* tail;
int length = 1;
for (tail = head; tail->next; tail = tail->next)
++length;
tail->next = head; // Circle the list.
const int t = length - k % length;
for (int i = 0; i < t; ++i)
tail = tail->next;
ListNode* newHead = tail->next;
tail->next = nullptr;
return newHead;
}
};
/* code provided by PROGIEZ */
61. Rotate List LeetCode Solution in Java
class Solution {
public ListNode rotateRight(ListNode head, int k) {
if (head == null || head.next == null || k == 0)
return head;
int length = 1;
ListNode tail = head;
for (; tail.next != null; tail = tail.next)
++length;
tail.next = head; // Circle the list.
final int t = length - k % length;
for (int i = 0; i < t; ++i)
tail = tail.next;
ListNode newHead = tail.next;
tail.next = null;
return newHead;
}
}
// code provided by PROGIEZ
61. Rotate List LeetCode Solution in Python
class Solution:
def rotateRight(self, head: ListNode, k: int) -> ListNode:
if not head or not head.next or k == 0:
return head
tail = head
length = 1
while tail.next:
tail = tail.next
length += 1
tail.next = head # Circle the list.
t = length - k % length
for _ in range(t):
tail = tail.next
newHead = tail.next
tail.next = None
return newHead
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.